1. React Component Element Rendering
class CorrectComponent extends React.Component {
// React Component is rendering only a single element.
render() {
return (
<h4> Hello React </h4>
)
}
}
class WrongComponent extends React.Component {
// React Component is not rendering more than one element.
render() {
return (
<h4> Hello </h4>
<h4> React </h4>
)
}
}
React Component renders React elements using the render
function, but it can only render a single element at a time. If more than one element is rendered, an error will occur.
Of course, there are ways to render multiple React elements at once.
Using Parent Tag
class MultiElementsComponent extends React.Component {
//
render() {
return (
<div>
<h4> Hello </h4>
<h4> React </h4>
</div>
)
}
}
In this case, the two tags are wrapped as child elements of a single parent tag (<div>
). Therefore, only one tag (React Element) is rendered, and no error occurs.
Using an Array
class ArrayElementComponent extends React.Component {
//
render() {
return [
<h4> Hello </h4>,
<h4> React </h4>
]
}
}
Starting from React version 16
, you can return an array in the render
function of a React Component. Multiple tags (React elements) can be returned as an array, separated by commas.
Using React.Fragment
class UseFragmentComponent extends React.Component {
//
render() {
return (
<React.Fragment>
<h4> Hello </h4>
<h4> React </h4>
</React.Fragment>
)
}
}
React.Fragment
is used to group multiple elements without adding them to the DOM. At first glance, it may look similar to using a parent tag to wrap the child elements, but the key difference is that React.Fragment
does not add any extra elements to the DOM.
Let’s see how this difference is applied.
2. The Introduction of React.Fragment
Let’s first create a component that generates a table.
class StudentInfo extends React.Component {
//
render() {
return (
<table>
<tr>
<th> Name </th>
<th> Age </th>
<th> Location </th>
</tr>
<tr>
<td> Terry </td>
<td> 24 </td>
<td> Seoul </td>
</tr>
</table>
)
}
}

We can split this table into the header part and the body part as components.
class TableHeader extends React.Component {
//
render() {
return (
<th> Name </th>
<th> Age </th>
<th> Location </th>
)
}
}
class TableBody extends React.Component {
//
render() {
return (
<td> Terry </td>
<td> 24 </td>
<td> Seoul </td>
)
}
}
Of course, both of these components must return only one element at a time, so we need to modify the code using one of the methods we discussed earlier.
First, let’s modify the code using a parent tag.
class TableHeader extends React.Component {
//
render() {
return (
<div>
<th> Name </th>
<th> Age </th>
<th> Location </th>
</div>
)
}
}
class TableBody extends React.Component {
//
render() {
return (
<div>
<td> Terry </td>
<td> 24 </td>
<td> Seoul </td>
</div>
)
}
}
Using the TableHeader
and TableBody
components to create a complete table.
class StudentInfo extends React.Component {
//
render() {
return (
<table>
<tr>
<TableHeader />
</tr>
<tr>
<TableBody />
</tr>
</table>
)
}
}

Does the table created initially give the same result as the table created by splitting components?
Probably not. This is because the tags within the table elements can lead to the creation of an unintended table. To solve this issue, we can use React.Fragment
. Let's modify the TableHeader
and TableBody
components we created earlier using React.Fragment
.
class TableHeader extends React.Component {
//
render() {
return (
<React.Fragment>
<th> Name </th>
<th> Age </th>
<th> Location </th>
</React.Fragment>
)
}
}
class TableBody extends React.Component {
//
render() {
return (
<React.Fragment>
<td> Terry </td>
<td> 24 </td>
<td> Seoul </td>
</React.Fragment>
)
}
}

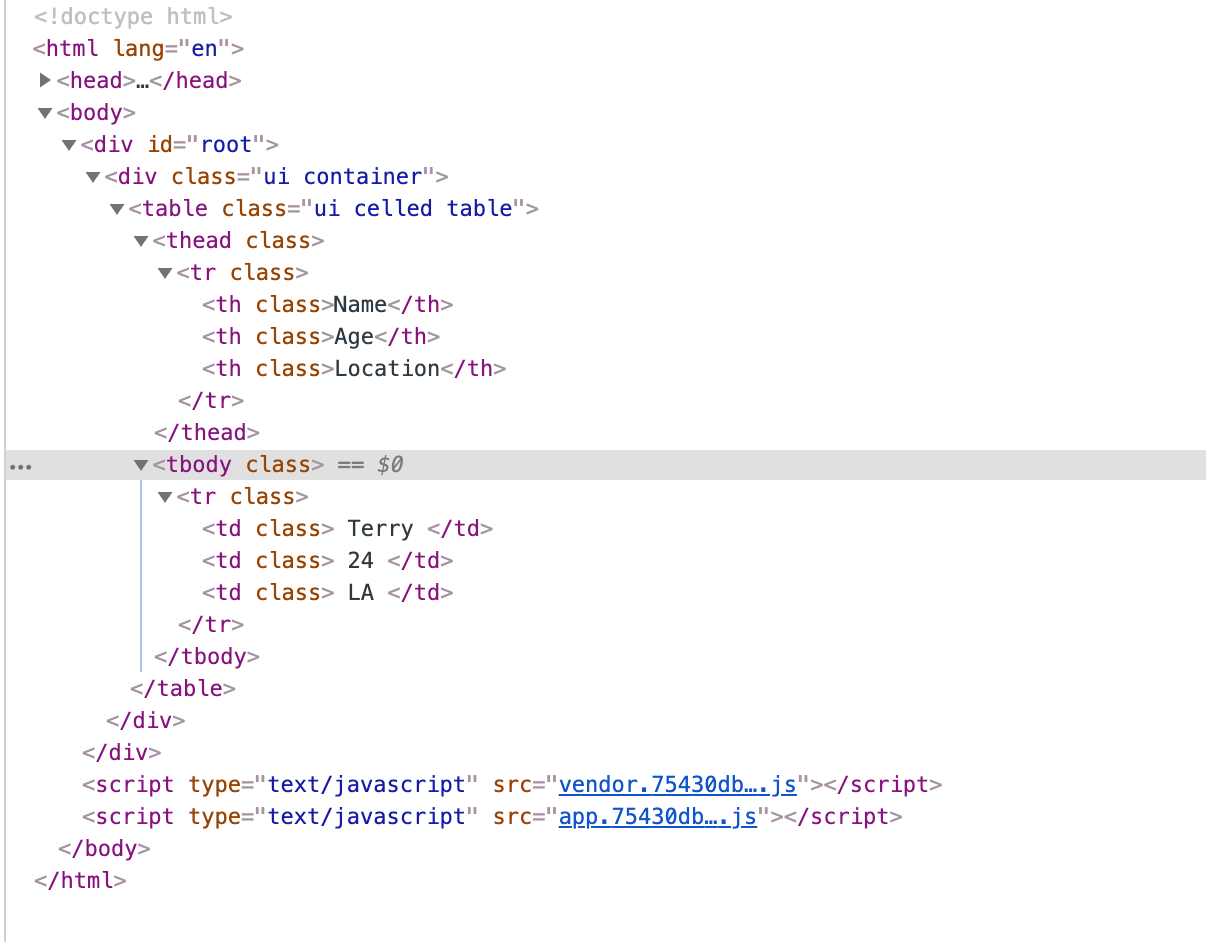
Now, we can see that the table created is the same as the initially created table.
Since React.Fragment
does not add elements to the DOM, we were able to create the intended table.
3. Additionally...
React.Fragment
can also be used with the shorthand syntax <>
instead of the full <React.Fragment>
tag.
class EmptyTag extends React.Component {
//
render() {
return (
<>
<h4> Hello </h4>
<h4> React </h4>
</>
)
}
}
However, since not many browsers support this shorthand syntax yet, it is recommended to use <React.Fragment>
for better compatibility. Additionally, React.Fragment
can also be rendered with a key
attribute.
In the future, React plans to add more event handlers that can be used with React.Fragment
. Thank you!
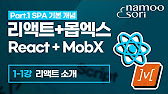